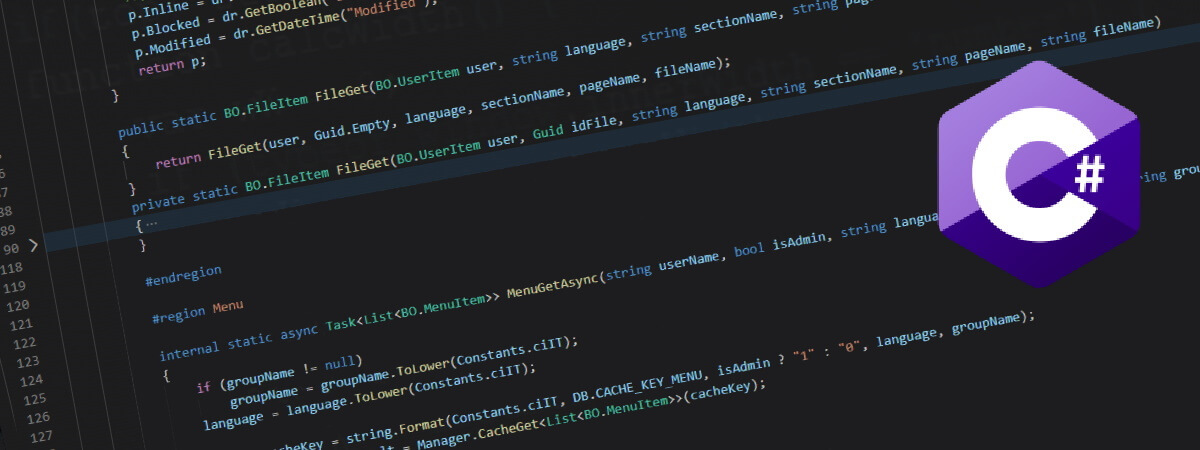
Combo in cascata con JQuery e WebService in ASP.NET
In questo esempio è possibile vedere come caricare delle combo dinamicamente. Per far questo uso JQuery e le chiamate AJAX per recupperare i dati da un Web Service scritto in ASP.NET che ritorna i dati necessari in formato XML.
Ecco in pratica l'esempio:
Per realizzare l'applicazione serve una pagina html con le combo e il codice JavaScript che, tramite JQuery, esegue le chiamate AJAX per recuperare i dati con cui riempire le combo Regioni, Province e Comuni.
Le cose interessanti che si possono vedere nell'esempio relative a JQuery sono:
Per quanto riguarda la pagina che restituisce i dati, ho usato una Web Service scritto in ASP.NET utilizzando C# facendomi restituire un DataSet che viene serializzato in XML.
I dati ritornati sono nel seguente formato:
Il Web Service che ritorna i dati è il seguente:
Se compare un errrore del tipo:
Ecco in pratica l'esempio:
Per realizzare l'applicazione serve una pagina html con le combo e il codice JavaScript che, tramite JQuery, esegue le chiamate AJAX per recuperare i dati con cui riempire le combo Regioni, Province e Comuni.
HTML
<form action="" method="post" id="SgartComuni" onsubmit="return false;">
<table>
<tr>
<td>Regione</td>
<td><select id="sgart_R" style="width:200px"><option>...</option></select></td>
</tr>
<tr>
<td>Province</td>
<td><select id="sgart_P" style="width:300px"><option>...</option></select></td>
</tr>
<tr>
<td>Comuni</td>
<td><select id="sgart_C" style="width:300px"><option>...</option></select></td>
</tr>
</table>
</form>
JavaScript
<script language="javascript">
$(document).ready(function(){
$("#sgart_R").change(sgart_loadProvince);
$("#sgart_P").change(sgart_loadComuni);
sgart_loadRegioni();
});
function sgart_loadRegioni() {
var codRegione = $("#sgart_R option:selected").val();
$("#sgart_R").html("<option>Attendi...</option>");
$("#sgart_P").html("<option></option>");
$("#sgart_C").html("<option></option>");
$.ajax({
type: "POST",
url: "/Utility/WS.asmx/SelectRegioni",
dataType: "xml",
success: function(xml) {
var rows = $(xml).find("Regioni").find("Table");
var r = "<option value=''></option>";
for (var i = 0; i < rows.length; i++) {
var code = $(rows[i]).find("CodRegione").text();
var description = $(rows[i]).find("Regione").text();
r += "<option value=\"" + code + "\">" + description + "</option>\r\n";
}
$("#sgart_R").html(r);
},
error: function(request, status, message){
alert(status + ": " + request.statusText);
}
});
}
function sgart_loadProvince() {
var code= $("#sgart_R option:selected").val();
$("#sgart_P").html("<option>Attendi...</option>");
$("#sgart_C").html("<option></option>");
$.ajax({
type: "POST",
url: "/Utility/WS.asmx/SelectProvince",
data: {codRegione : code},
dataType: "xml",
success: function(xml) {
var rows = $(xml).find("Province").find("Table");
var r = "<option value=''></option>";
for (var i = 0; i < rows.length; i++) {
var code = $(rows[i]).find("CodProvincia").text();
var description = $(rows[i]).find("Provincia").text();
r += "<option value=\"" + code + "\">" + description + "</option>\r\n";
}
$("#sgart_P").html(r);
},
error: function(request, status, message){
alert(status + ": " + request.statusText);
}
});
}
function sgart_loadComuni() {
var code= $("#sgart_P option:selected").val();
$("#sgart_C").html("<option>Attendi...</option>");
$.ajax({
type: "POST",
url: "/Utility/WS.asmx/SelectComuni",
data: {codProvincia : code},
dataType: "xml",
success: function(xml) {
var rows = $(xml).find("Comuni").find("Table");
var r = "<option value=''></option>";
for (var i = 0; i < rows.length; i++) {
var code = $(rows[i]).find("ID").text();
var description = $(rows[i]).find("Comune").text();
r += "<option value=\"" + code + "\">" + description + "</option>\r\n";
}
$("#sgart_C").html(r);
},
error: function(request, status, message){
alert(status + ": " + request.statusText);
}
});
}
</script>
- come chiamare un Web Service $.ajax({ type, url, data, dataType, success, error });
- come richiamare un metodo del Web Service nel paramentro url componendo la url come path/paginaWebService/nomeMetodo
- come passare dei parametri al Web Service nel paramtro data come { nomeParamentro : valoreParametro }
- come recuperare un nodo dell'xml di riposta: $(xml).find("Regioni").find("Table")
- come recuperare il valore di un nodo $(rows[i]).find("CodRegione").text()
Per quanto riguarda la pagina che restituisce i dati, ho usato una Web Service scritto in ASP.NET utilizzando C# facendomi restituire un DataSet che viene serializzato in XML.
I dati ritornati sono nel seguente formato:
XML
<?xml version="1.0" encoding="utf-8"?>
<DataSet xmlns="http://www.sgart.it/utility/">
<xs:schema id="Regioni" xmlns="" xmlns:xs="http://www.w3.org/2001/XMLSchema" xmlns:msdata="urn:schemas-microsoft-com:xml-msdata">
<xs:element name="Regioni" msdata:IsDataSet="true" msdata:UseCurrentLocale="true">
<xs:complexType>
<xs:choice minOccurs="0" maxOccurs="unbounded">
<xs:element name="Table">
<xs:complexType>
<xs:sequence>
<xs:element name="CodRegione" type="xs:string" minOccurs="0" />
<xs:element name="Regione" type="xs:string" minOccurs="0" />
</xs:sequence>
</xs:complexType>
</xs:element>
</xs:choice>
</xs:complexType>
</xs:element>
</xs:schema>
<diffgr:diffgram xmlns:msdata="urn:schemas-microsoft-com:xml-msdata" xmlns:diffgr="urn:schemas-microsoft-com:xml-diffgram-v1">
<Regioni xmlns="">
<Table diffgr:id="Table1" msdata:rowOrder="0">
<CodRegione>ABR</CodRegione>
<Regione>Abruzio</Regione>
</Table>
<Table diffgr:id="Table2" msdata:rowOrder="1">
<CodRegione>BAS</CodRegione>
<Regione>Basilicata</Regione>
</Table>
</Regioni>
</diffgr:diffgram>
</DataSet>
HTML: WS.asmx
<%@ WebService Language="C#" CodeBehind="~/App_Code/WS.cs" Class="WS" %>
C#: /App_Code/WS.asmx.cs
using System;
using System.Collections.Generic;
using System.Web;
using System.Web.Services;
using System.Configuration;
using System.Data;
using System.Data.OleDb;
/// <summary>
/// Summary description for ws
/// </summary>
[WebService(Namespace = "http://www.sgart.it/utility/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
public class WS : System.Web.Services.WebService
{
#region SELECT
[WebMethod(Description = "Return a dataset with Regioni")]
public DataSet SelectRegioni()
{
DataSet ds = new DataSet("Regioni");
try
{
if (!IsValidOriginRequest) return null;
using (OleDbConnection cnn = GetConnection())
{
using (OleDbCommand cmd = cnn.CreateCommand())
{
cmd.CommandType = CommandType.Text;
cmd.CommandText = @"SELECT [CodRegione], [Regione]
FROM [TabRegioni]
ORDER BY [Regione]";
using (OleDbDataAdapter da = new OleDbDataAdapter(cmd))
{
da.Fill(ds);
}
}
}
}
catch (Exception ex)
{
Context.Response.StatusCode = 500;
Context.Response.StatusDescription = "Regioni, " +ex.Message;
}
return ds;
}
[WebMethod(Description = "Return a dataset with Province")]
public DataSet SelectProvince(string codRegione)
{
DataSet ds = new DataSet("Province");
try
{
if (!IsValidOriginRequest) return null;
using (OleDbConnection cnn = GetConnection())
{
using (OleDbCommand cmd = cnn.CreateCommand())
{
cmd.CommandType = CommandType.Text;
cmd.CommandText = @"SELECT [CodProvincia], [Provincia]
FROM [TabProvince]
WHERE [CodRegione] = ?
ORDER BY [Provincia]";
OleDbParameter param = cmd.Parameters.Add("codRegione", OleDbType.VarChar, 4);
param.Value = codRegione;
using (OleDbDataAdapter da = new OleDbDataAdapter(cmd))
{
da.Fill(ds);
}
}
}
}
catch (Exception ex)
{
Context.Response.StatusCode = 500;
Context.Response.StatusDescription = "Province, " +ex.Message;
}
return ds;
}
[WebMethod(Description = "Return a dataset with Comuni")]
public DataSet SelectComuni(string codProvincia)
{
DataSet ds = new DataSet("Comuni");
try
{
if (!IsValidOriginRequest) return null;
using (OleDbConnection cnn = GetConnection())
{
using (OleDbCommand cmd = cnn.CreateCommand())
{
cmd.CommandType = CommandType.Text;
cmd.CommandText = @"SELECT [ID], [Comune], [CAP], [PrefissoTelefonico]
, [CodErariale], [CodISTAT]
FROM [TabComuni]
WHERE [CodProvincia] = ?
ORDER BY [Comune]";
OleDbParameter param = cmd.Parameters.Add("CodProvincia", OleDbType.VarChar, 4);
param.Value = codProvincia;
using (OleDbDataAdapter da = new OleDbDataAdapter(cmd))
{
da.Fill(ds);
}
}
}
}
catch (Exception ex)
{
Context.Response.StatusCode = 500;
Context.Response.StatusDescription = "Comuni, " + ex.Message;
}
return ds;
}
#endregion
#region Private method
private OleDbConnection GetConnection()
{
string connectionString = ConfigurationManager.ConnectionStrings["cnnAccess"].ConnectionString.Replace("~", Server.MapPath("/"));
return new OleDbConnection(connectionString);
}
public bool IsValidOriginRequest
{
get
{
string referer = "";
try
{
referer = Context.Request.UrlReferrer.Host;
}
catch { }
return Context.Request.IsLocal == true || referer.Equals(Context.Request.Url.Host, StringComparison.InvariantCultureIgnoreCase);
}
}
#endregion
}
Request format is unrecognized for URL unexpectedly ending in /myMethodName
aggiungere al web.config:XML: web.config
<configuration>
<system.web>
<webServices>
<protocols>
<add name="HttpGet"/>
<add name="HttpPost"/>
</protocols>
</webServices>
</system.web>
</configuration>