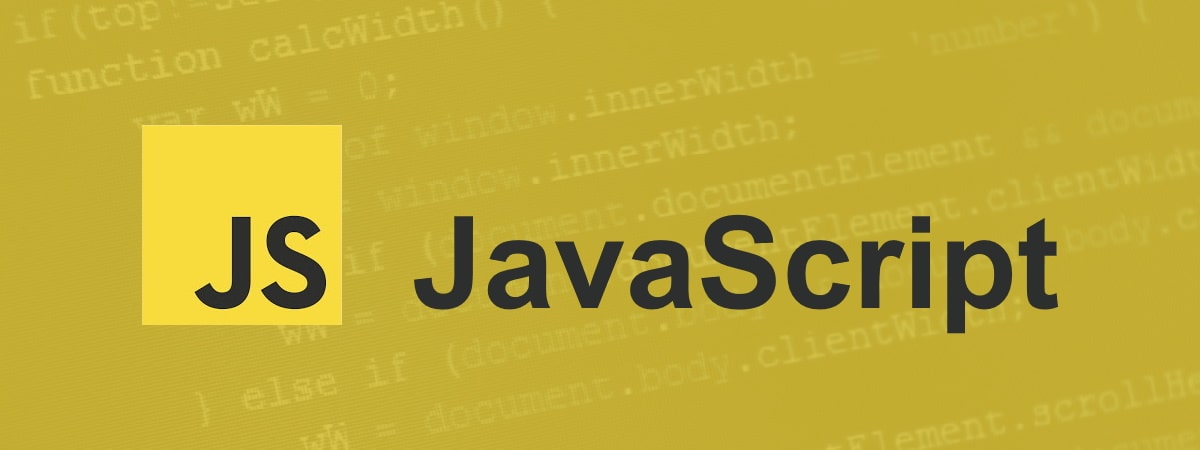
Funzione isNullOrWhiteSpace in JavaScript
In C# esiste un utile funzione string.isNullOrWhiteSpace(s) che permette di verificare se una stringa è nulla, vuota o di lunghezza zero senza considerare gli spazi iniziali e finali (Trim).
In questa condizione ritorna true, mentre se è una stringa valida, ovvero non composta da soli spazi, ritorna false.
Può essere scritta in forma semplificata come arrow function
oppure come modulo NodeJs
esempio in JavaScript:
esempio in NodeJs
In questa condizione ritorna true, mentre se è una stringa valida, ovvero non composta da soli spazi, ritorna false.
Implementazione
E' possibile implementare la stessa funzione in JavaScriptJavaScript
var sgart = sgart || {};
sgart.isNullOrWhiteSpace = function (v) {
if (v === undefined || v === null || v.length === 0) return true;
if (typeof v !== "string") return true;
return v.trim().length === 0;
};
La funzione verifica anche che la variabile non sia undefined e che sia di tipo string.
Può essere scritta in forma semplificata come arrow function
JavaScript
sgart.isNullOrWhiteSpace = (v) => {
return null == s || 0 === s.length || ('string' != typeof s || 0 === s.trim().length);
};
Demo
Esempio:JavaScript
var s = undefined;
sgart.isNullOrWhiteSpace(s); // true
var s = null;
sgart.isNullOrWhiteSpace(s); // true
var s = " ";
sgart.isNullOrWhiteSpace(s); // true
var s = " d ";
sgart.isNullOrWhiteSpace(s); // false
var s = " d ";
sgart.isNullOrWhiteSpace(s); // true
Variante
Volendo è possibile fare una variante per generare un eccezione nel caso il valore non sia di tipo stringJavaScript
sgart.isNullOrWhiteSpace = function (v) {
if (v === undefined || v === null) return true;
if (typeof v !== "string") throw 'Invalid type';
return v.trim().length === 0;
};
JavaScript: helpers/helper.js
/**
* Verifica se una stringa è undefined, null, empty (lunghezza 0 spazi esclusi)
* @param {string} s
* @returns
*/
const isNullOrWhiteSpace = (s) => {
if('string' !== typeof s) throw 'Invalid type';
return null == s || 0 === s.length || 0 === s.trim().length;
};
module.exports = {
isNullOrWhiteSpace
};
JavaScript
var s = new Date();
sgart.isNullOrWhiteSpace(s); // Uncaught Invalid type
JavaScript
const helper = require('../helpers/helper');
const s = " xx";
const empty = helper.isNullOrWhiteSpace(s);
Alternativa Typescript
In alternativa la funzione può essere scritta in TypeScript in questo modoTypeScript
export const isNullOrWhiteSpace = (str: string): boolean => {
//if ('string' !== typeof str) throw 'Invalid type';
if (undefined === str || null === str) return true;
if ((/^\s*$/g).test(str)) return true;
return false;
};