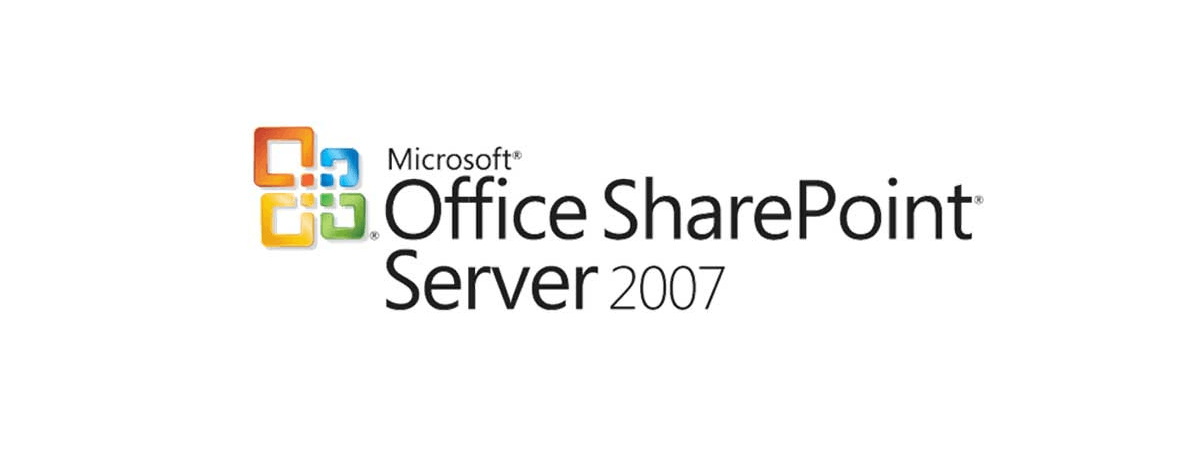
Aggungere dei permission level e gruppi ad un sito
In questo esempio si vede come in SharePoint 2007 (WSS3 - MOSS) è possibile aggiungere dei nuovi permission level (RoleDefinitions), dei nuovi gruppi (SPGroup) ed associare il gruppo con uno specifico permission level (SPRoleAssignment) ed aggiungerlo ad un sito (RoleDefinitionBindings). Infine vengono aggiunti degli utenti al gruppo (metodo AddUser);
Nell'esempio viene aggiunto tutto al sito root della site collection (SPSite), ma è possibile fare lo stesso anche negli altri siti a patto che sia spezzata l'ereditarietà. Inoltre il gruppo va sempre creato sulla site collection (webRoot.SiteGroups) e poi associato a un altro sito (SPWeb).
C#
//using Microsoft.SharePoint;
//add a perission level and group to site collection
string siteUrl = "http://<servername>";
using (SPSite site = new SPSite(siteUrl))
{
using (SPWeb webRoot = site.RootWeb)
{
//----------------------------------------------
//create a new permission level, if don't exist
string permissionName = "SgartPermission";
SPRoleDefinition perm = null;
try
{
//check if already exists
perm = webRoot.RoleDefinitions[permissionName];
}
catch { }
if (perm == null)
{
perm = new SPRoleDefinition();
perm.Name = permissionName;
perm.Description = "Example of permission added by code";
perm.BasePermissions = SPBasePermissions.ViewListItems
| SPBasePermissions.EditListItems;
//add it to root of site collection
webRoot.RoleDefinitions.Add(perm);
}
//----------------------------------------------
// create a new group in site collection
string groupName = "SgartGroup";
SPGroup group = null;
try
{
//check if group exists
group = webRoot.SiteGroups[groupName];
}
catch
{
//add a group to site collection
SPUser groupOwner = webRoot.CurrentUser;
webRoot.SiteGroups.Add(groupName, groupOwner, null, "New group added by code");
group = webRoot.SiteGroups[groupName];
// create a new role Assignment
SPRoleAssignment ra = new SPRoleAssignment(group);
// add the permission level 'SgartPermission' to group
ra.RoleDefinitionBindings.Add(perm);
// add the new role assignment
webRoot.RoleAssignments.Add(ra);
//add user to group
group.AddUser(groupOwner);
group.AddUser(webRoot.SiteAdministrators[0]);
}
}
}