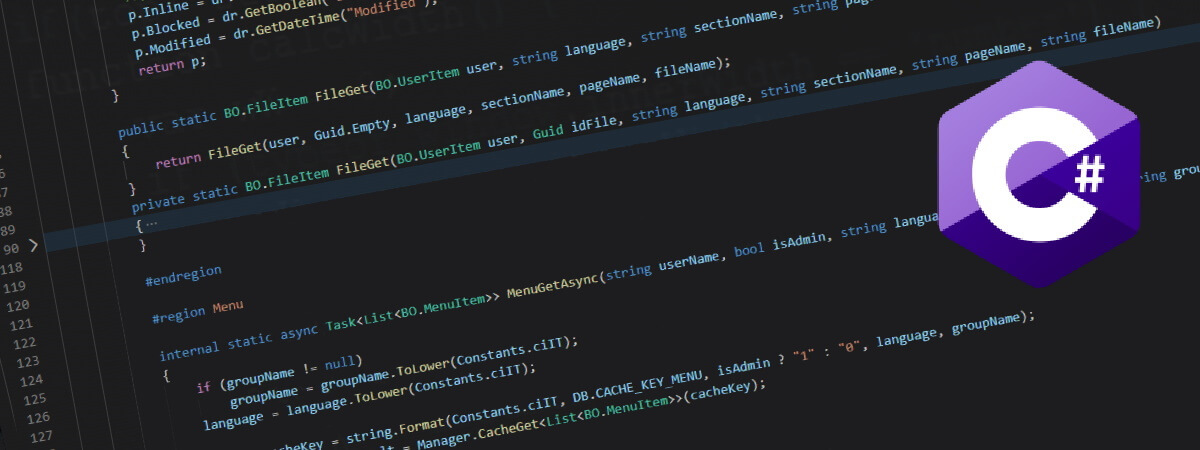
Come trovare le coordinate di un indirizzo usando maps.google.it
Un esempio C# su come trovare le coordinate geografiche di un indirizzo usando il sito http://maps.google.it.
Costruisco una url con l'indirizzo nella forma
e poi faccio il parse della pagina per trovare le coordinate.
Queste sono nella forma:
Costruisco una url con l'indirizzo nella forma
Text
http://maps.google.it/maps?q=Via+farini,+10,+Milano&hl=it
Queste sono nella forma:
Text
ssl=00.0000,00.00000
C#
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Net;
using System.Text.RegularExpressions;
namespace GoogleMapsResolveAddress
{
class Program
{
static void Main(string[] args)
{
string address = "Via+farini,+10,+Milano";
double lat = -1000;
double lon = -1000;
bool resolved= ResolveAddress(address, out lat, out lon);
if (resolved)
{
Console.WriteLine(string.Format("{1},{2} -> {0}", address, lat, lon));
}
else
{
Console.WriteLine("NOT FOUND");
}
}
private static bool ResolveAddress(string address, out double lat, out double lon)
{
lat = -1000;
lon = -1000;
bool resolved = false;
string url = string.Format("http://maps.google.it/maps?q={0}&hl=it", address);
using (WebClient wc = new WebClient())
{
wc.Credentials = System.Net.CredentialCache.DefaultCredentials;
//download page content
string content = wc.DownloadString(url);
/*<a id="link" jsaction="link.show" title="Link"
* href="http://maps.google.it/maps?q=Via+Farini,+1,+Milano&hl=it&ie=UTF8&hq=&hnear=Via+Farini,+1,+Milano,+Lombardia&sll=45.636307,9.166868&spn=0.003571,0.016716&t=m&z=16&vpsrc=0"
* class="kd-button permalink-button right small" jstcache="0">
* <img class="link" src="//maps.gstatic.com/mapfiles/transparent.png" jstcache="0">
* </a>
* */
System.Globalization.CultureInfo ciEn = new System.Globalization.CultureInfo(1033);
Regex reCoords = new Regex(@"sll=(?<lat>[0-9]*\.[0-9]*),(?<lon>[0-9]*\.[0-9]*)"
, RegexOptions.IgnoreCase | RegexOptions.Singleline | RegexOptions.ExplicitCapture);
//find all href
Regex re = new Regex(@"href=(""|')(.+?)(\1)", RegexOptions.IgnoreCase | RegexOptions.Multiline);
foreach (Match item in re.Matches(content))
{
if (item.Value.Contains(address) && item.Value.Contains("sll="))
{
//find: sll=45.636307,9.166868
Match m = reCoords.Match(item.Value);
if (m.Success)
{
//parse coords
double.TryParse(m.Groups["lat"].Value, System.Globalization.NumberStyles.AllowDecimalPoint, ciEn, out lat);
double.TryParse(m.Groups["lon"].Value, System.Globalization.NumberStyles.AllowDecimalPoint, ciEn, out lon);
resolved = true;
break;
}
}
}
return resolved;
}
}
}
}