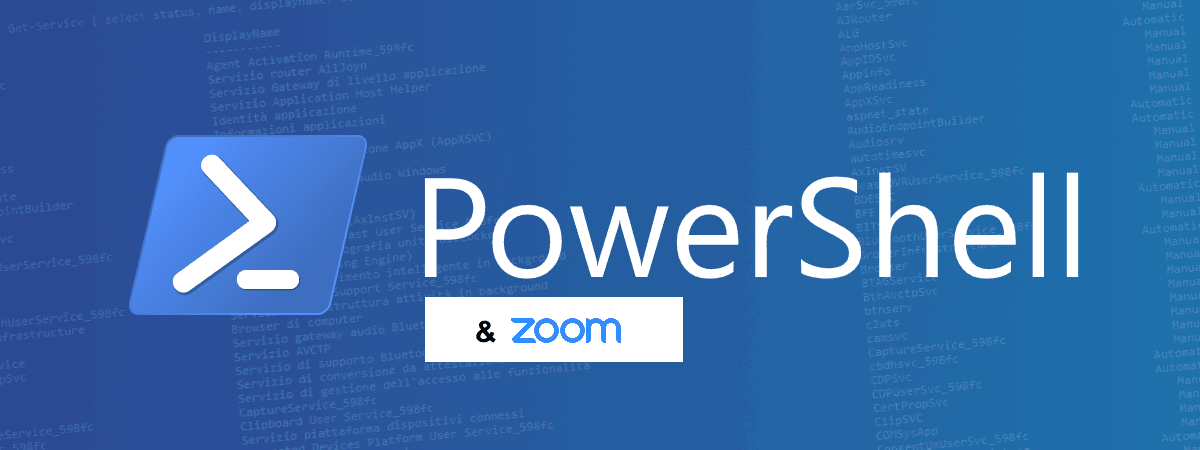
Creare un meeting Zoom da PowerShell
Tramite PowerShell è possibile automatizzare la creazione di meeting Zoom tramite lo script seguente.
Per prima cosa va creata una App di tipo JWT dal marketplace di Zoom ( https://marketplace.zoom.us/develop/create )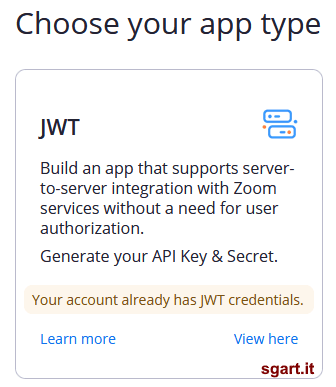
Creazione App di tipo JWT Una volta creata la app vengono mostrati i valori API Key e API Secret generati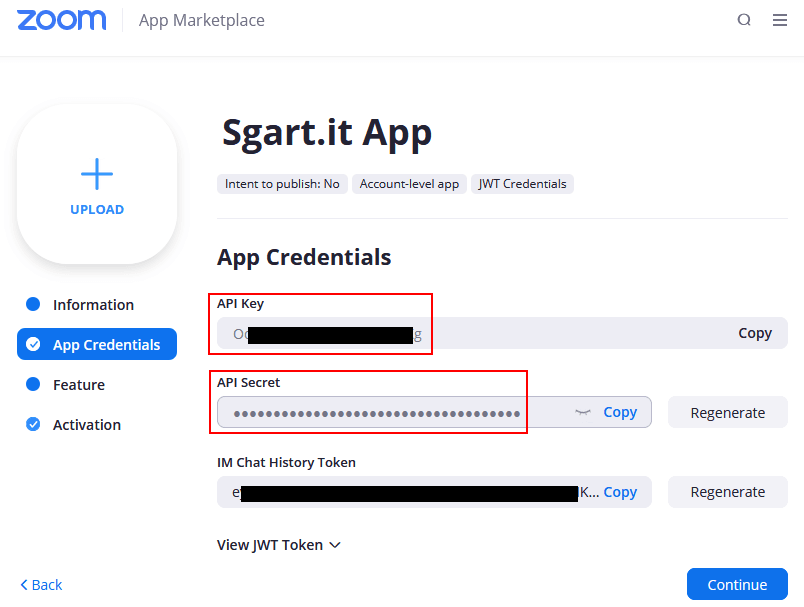
API Key e Secret
Lo script va inizializzato con i valori di API Key e API Secret ($apiKey e $apiSecret), con la durata desiderata del token JWT espressa in secondi ($tokenDurationSeconds) infine imposto la url base dell chiamate alle API ($apiBaseUrl)
Il funzionamento dello script richiede l'installazione sulla macchina di alcuni pacchetti NuGet, nello specifico System.IdentityModel.Tokens.Jwt.
La seguente funzione automatizza il download di nuget.exe e poi scarica e installa il pacchetto necessario, infine aggiunge i tipi richiesti (Add-Type)
Con le librerie installate è possibile generare il token JWT usando i valori di API Key e API Secret tramite la seguente funzione GetJwtToken
L'ultima funzione necessaria è PostData che esegue una chiamate REST in POST inviando l'oggetto passato serializzato in JSON
Adesso ho il necessario per costruire un oggetto che rappresenta il meeting ($meeting) ed eseguire la chiamata alla API
La chiamata restituisce un JSON che rappresenta il meeting appena chreato
dove, id è l'identificati univoco assegnato al mmeting, la start_url è il link da usare per da parte del proprietario della riunione e join_url e la url, unica, da inviare a tutti gli utenti senza la necessità che si registrino.
Per i dettagli sui parametri da passare e i valori di ritorno vedi POST /users/{userId}/meetings
Per prima cosa va creata una App di tipo JWT dal marketplace di Zoom ( https://marketplace.zoom.us/develop/create )
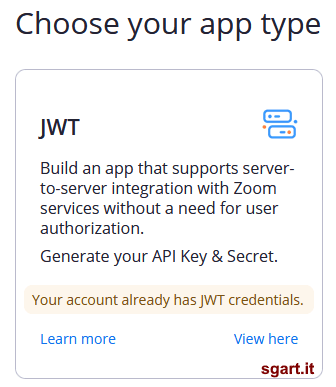
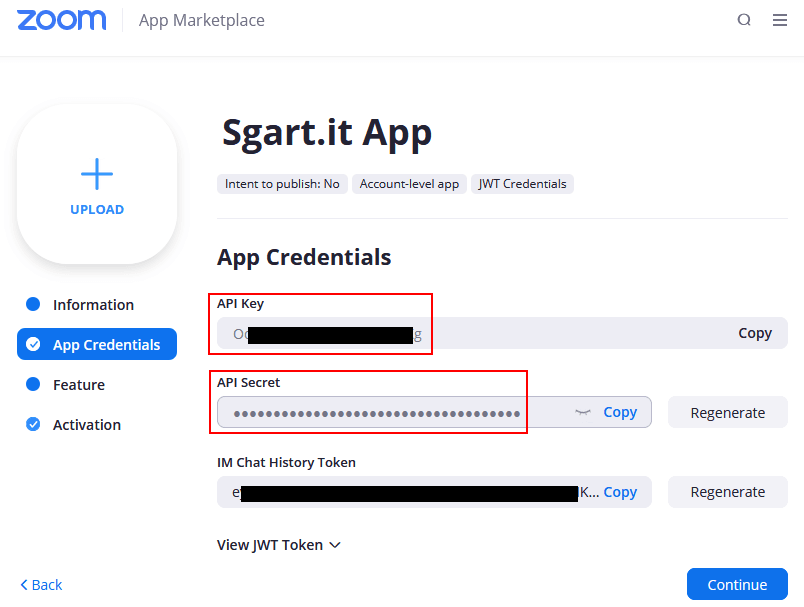
Lo script va inizializzato con i valori di API Key e API Secret ($apiKey e $apiSecret), con la durata desiderata del token JWT espressa in secondi ($tokenDurationSeconds) infine imposto la url base dell chiamate alle API ($apiBaseUrl)
PowerShell
<#
Sgart.it
https://www.sgart.it/IT/informatica/creare-un-meeting-zoom-da-powershell/post
#>
$apiKey = "Oo.......Xg";
$apiSecret = "zv2n1........eSb";
$tokenDurationSeconds = 300;
$apiBaseUrl = "https://api.zoom.us/v2";
La seguente funzione automatizza il download di nuget.exe e poi scarica e installa il pacchetto necessario, infine aggiunge i tipi richiesti (Add-Type)
PowerShell
<#
Carica i tipi necessari
prima verifica se è installato il pacchetto
se non riesce, si assicura che esista nuget.exe,
poi prova a scaricare ed installare il relativo package nuget
#>
function LoadLibraries {
$jwtLibVersion = "6.8.0"; # versione della libreria
if ($PSVersionTable.PSEdition -eq 'Desktop') {
$edition = "net45";
}
else {
$edition = "netstandard2";
}
$nugetPackages = "$env:USERPROFILE\.nuget\packages";
if($false -eq (Test-Path "$nugetPackages\System.IdentityModel.Tokens.Jwt\$jwtLibVersion\lib\$edition")) {
# se non trovo il pacchetto lo installo
$downloadFolder = "$env:USERPROFILE\Downloads";
$nugetExe = "$downloadFolder\nuget.exe"
if($false -eq (Test-Path $nugetExe) ){
# Scarica l'ultima versione di nuget.exe se non è già presente
Write-Host "Dowloading nuget.exe ..."
Invoke-WebRequest -Uri 'https://dist.nuget.org/win-x86-commandline/latest/nuget.exe' -OutFile "$nugetExe";
}
# Scarica e installa il pacchetto System.IdentityModel.Tokens.Jwt
# e le sue dipendenze: Microsoft.IdentityModel.JsonWebTokens, Microsoft.IdentityModel.Logging e Microsoft.IdentityModel.Tokens
Write-Host "Dowloading and installing System.IdentityModel.Tokens.Jwt ..."
& "$nugetExe" Install System.IdentityModel.Tokens.Jwt -Version $jwtLibVersion -OutputDirectory "$downloadFolder";
}
# aggiungo tipi necessari
Add-Type -Path "$nugetPackages\Microsoft.IdentityModel.Tokens\$jwtLibVersion\lib\$edition\Microsoft.IdentityModel.Tokens.dll";
Add-Type -Path "$nugetPackages\System.IdentityModel.Tokens.Jwt\$jwtLibVersion\lib\$edition\System.IdentityModel.Tokens.Jwt.dll";
Add-Type -Path "$nugetPackages\Microsoft.IdentityModel.Logging\$jwtLibVersion\lib\$edition\Microsoft.IdentityModel.Logging.dll";
Add-Type -Path "$nugetPackages\Microsoft.IdentityModel.JsonWebTokens\$jwtLibVersion\lib\$edition\Microsoft.IdentityModel.JsonWebTokens.dll";
Add-Type -AssemblyName System.Text.Encoding;
Add-Type -AssemblyName System.IdentityModel;
}
Testato con il .Net Framework 4.8 installato sulla macchina.
Con le librerie installate è possibile generare il token JWT usando i valori di API Key e API Secret tramite la seguente funzione GetJwtToken
PowerShell
<#
Crea il token JWT partendo da ApiKey e ApiSecret
#>
function GetJwtToken {
$tokenHandler = New-Object System.IdentityModel.Tokens.Jwt.JwtSecurityTokenHandler;
[byte[]]$symmetricKey = [System.Text.Encoding]::ASCII.GetBytes($apiSecret);
$symmetricSecurityKey = New-Object Microsoft.IdentityModel.Tokens.SymmetricSecurityKey(@(, $symmetricKey));
$SigningCredentials = New-Object Microsoft.IdentityModel.Tokens.SigningCredentials(
$symmetricSecurityKey,
[Microsoft.IdentityModel.Tokens.SecurityAlgorithms]::HmacSha256
);
$tokenDescriptor = New-Object Microsoft.IdentityModel.Tokens.SecurityTokenDescriptor;
$tokenDescriptor.Issuer = $apiKey;
$tokenDescriptor.Expires = (Get-Date).AddSeconds($tokenDurationSeconds);
$tokenDescriptor.SigningCredentials = $SigningCredentials;
$token = $tokenHandler.CreateToken($tokenDescriptor);
$strToken = $tokenHandler.WriteToken($token);
Write-Host "JWT: $strToken"
$strToken
}
L'ultima funzione necessaria è PostData che esegue una chiamate REST in POST inviando l'oggetto passato serializzato in JSON
PowerShell
<#
Post di un generico oggetto in formato JSON
#>
function PostData {
param (
[string] $apiRelativeUrl,
[object] $data
)
$dataJson = $data | ConvertTo-Json;
# recupero il token JWT
$token = GetJwtToken
$headers = @{
"content-type" = "application/json";
"authorization" = "Bearer $token"
};
# effettuo la chiamata alla API aggiungendo il percorso base
$response = Invoke-RestMethod -Uri "$apiBaseUrl$apiRelativeUrl" -Method POST -Headers $headers -Body $dataJson;
$response;
}
Adesso ho il necessario per costruire un oggetto che rappresenta il meeting ($meeting) ed eseguire la chiamata alla API
URL
https://api.zoom.us/v2/users/me/meetings
PowerShell
# carico le libreire
LoadLibraries
# creo un meeting schedulato
$meeting = @{
topic = "Meeting PowerShell";
start_time = (Get-Date).AddMinutes(10).ToUniversalTime().ToString("yyyy-MM-ddTHH:mm:ssZ");
duration = 90; # in minuti
type = 2; # ScheduledMeeting
password = "231221"; # password da generare casualmente
settings = @{
waiting_room = $true;
}
};
# eseguo la chiamata alla API
$response = PostData "/users/me/meetings" $meeting
Write-Host ($response | ConvertTo-Json)
La chiamata restituisce un JSON che rappresenta il meeting appena chreato
JSON
{
"uuid": "pA4.......Ovi+g==",
"id": 9.........2,
"host_id": "-D_XYW......MoFA",
"host_email": "xxxx@dominio.it",
"topic": "Meeting PowerShell",
"type": 2,
"status": "waiting",
"start_time": "2021-01-27T23:05:27Z",
"duration": 90,
"timezone": "Europe/Rome",
"created_at": "2021-01-27T23:53:37Z",
"start_url": "https://zoom.us/s/9.........2?zak=ey********f5.ey**********xf.6v************d8",
"join_url": "https://zoom.us/j/9.........2?pwd=dm5Q..........ndz09",
"password": "231221",
"h323_password": "231221",
"pstn_password": "231221",
"encrypted_password": "dm5......09",
"settings": {
"host_video": false,
"participant_video": false,
"cn_meeting": false,
"in_meeting": false,
"join_before_host": false,
"jbh_time": 0,
"mute_upon_entry": false,
"watermark": false,
"use_pmi": false,
"approval_type": 2,
"audio": "both",
"auto_recording": "none",
"enforce_login": false,
"enforce_login_domains": "",
"alternative_hosts": "",
"close_registration": false,
"show_share_button": false,
"allow_multiple_devices": false,
"registrants_confirmation_email": true,
"waiting_room": true,
"request_permission_to_unmute_participants": false,
"global_dial_in_countries": [
"US"
],
"global_dial_in_numbers": [
"@{country_name=US; number=+1 25......82; type=toll; country=US}",
"@{country_name=US; number=+1 30......92; type=toll; country=US}",
"@{country_name=US; number=+1 31......99; type=toll; country=US}",
"@{country_name=US; number=+1 34......99; type=toll; country=US}",
"@{country_name=US; number=+1 66......33; type=toll; country=US}",
"@{country_name=US; number=+1 92......99; type=toll; country=US}"
],
"registrants_email_notification": true,
"meeting_authentication": false,
"encryption_type": "enhanced_encryption",
"approved_or_denied_countries_or_regions": {
"enable": false
}
}
}
Per i dettagli sui parametri da passare e i valori di ritorno vedi POST /users/{userId}/meetings