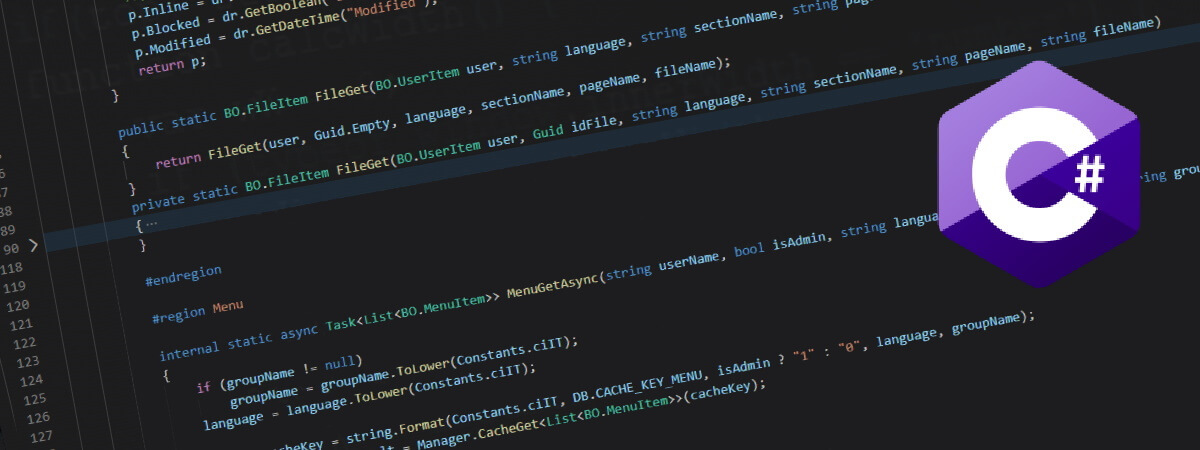
GridView editing e sorting
Un esempio di come è possibile con il .NET 2.0 gestire l'editing e il sorting della GridView in C#.
In questo caso, come data source, uso un DataTable memorizzato nel ViewState (vedi LoadDataSource)
Sono implementate le funzioni: insert, update, delete e sorting.
In questo caso, come data source, uso un DataTable memorizzato nel ViewState (vedi LoadDataSource)
Sono implementate le funzioni: insert, update, delete e sorting.
XML: Default.aspx
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default.aspx.cs" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title>GridView Editing example - Sgart.it</title>
</head>
<body>
<form id="form1" runat="server">
<h3>
GridView Editing example - Sgart.it</h3>
<asp:Panel ID="PnlGrid" runat="server">
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" CellPadding="2"
ForeColor="Black" GridLines="None"
BackColor="LightGoldenrodYellow" BorderColor="Tan" BorderWidth="1px"
DataKeyNames="ID"
OnRowDeleting="GridView1_RowDeleting"
OnRowEditing="GridView1_RowEditing"
OnRowUpdating="GridView1_RowUpdating"
OnRowCancelingEdit="GridView1_RowCancelingEdit"
OnSorting="GridView1_Sorting"
AllowSorting="True" >
<Columns>
<asp:BoundField DataField="Code" HeaderText="Codice" SortExpression="Code" />
<asp:BoundField DataField="Description" HeaderText="Descrizione" SortExpression="Description" />
<asp:BoundField DataField="Modified" HeaderText="Modificato il" ReadOnly="True" SortExpression="Modified" />
<asp:CommandField ShowEditButton="True" ShowDeleteButton="true" HeaderText="Edit" />
</Columns>
<FooterStyle BackColor="Tan" />
<PagerStyle BackColor="PaleGoldenrod" ForeColor="DarkSlateBlue" HorizontalAlign="Center" />
<SelectedRowStyle BackColor="DarkSlateBlue" ForeColor="GhostWhite" />
<HeaderStyle BackColor="Tan" Font-Bold="True" />
<AlternatingRowStyle BackColor="PaleGoldenrod" />
</asp:GridView>
</asp:Panel>
<asp:Panel ID="PnlButton" runat="server">
<asp:Button ID="BtnNew" runat="server" Text="Nuovo" OnClick="BtnNew_Click" />
<asp:Button ID="BtnRefresh" runat="server" Text="Refresh" OnClick="BtnRefresh_Click" />
<asp:Button ID="BtnReload" runat="server" Text="Reload" OnClick="BtnReload_Click" />
</asp:Panel>
<asp:Panel ID="PnlNew" runat="server">
<h4>
Insert</h4>
Code:
<asp:TextBox ID="TxtCode" runat="server"></asp:TextBox>
<br />
Description:
<asp:TextBox ID="TxtDescription" runat="server"></asp:TextBox>
<br />
<asp:Button ID="BtnNewSave" runat="server" OnClick="BtnNewSave_Click" Text="Salva" />
<asp:Button ID="BtnNewCancel" runat="server" Text="Annulla" OnClick="BtnNewCancel_Click" />
</asp:Panel>
</form>
</body>
</html>
C#: Default.aspx.cs
using System;
using System.Collections.Generic;
using System.Web;
using System.Web.UI;
using System.Web.UI.WebControls;
using System.Data;
public partial class _Default : System.Web.UI.Page
{
protected void Page_Load(object sender, EventArgs e)
{
if (!Page.IsPostBack)
{
LoadDataSource();
PnlNew.Visible = false;
}
}
#region Manage DataSource
//test data source
private DataTable Table
{
get { return (DataTable)ViewState["tbl"]; }
set { ViewState["tbl"] = value; }
}
private void LoadDataSource()
{
//bild example data source
DataTable tbl = new DataTable();
//add columns
DataColumnCollection cols = tbl.Columns;
cols.Add("ID", typeof(System.Int32));
cols.Add("Code", typeof(System.String));
cols.Add("Description", typeof(System.String));
cols.Add("Modified", typeof(System.DateTime));
//add rows
DataRowCollection rows = tbl.Rows;
rows.Add(new object[] { 2, "KJ7", "Piastra madre", DateTime.Now });
rows.Add(new object[] { 17, "FH5", "Processore", DateTime.Now });
rows.Add(new object[] { 4, "ER4", "Ram", DateTime.Now });
rows.Add(new object[] { 33, "W34", "Rom", DateTime.Now });
rows.Add(new object[] { 1, "SS2", "Hard Disk", DateTime.Now });
rows.Add(new object[] { 7, "SD3", "Video", DateTime.Now });
rows.Add(new object[] { 8, "TA9", "Tastiera", DateTime.Now });
Table = tbl;
Bind();
}
private void DataSourceUpdate(string code, string description)
{
DataSourceUpdate(0, code, description);
}
private void DataSourceUpdate(int id, string code, string description)
{
DataRow row;
if (id != 0)
{
DataRow[] rows = Table.Select(string.Format("ID={0}", id));
row = rows[0];
}
else
{
row = Table.NewRow();
Table.Rows.Add(row);
//get new id
row["ID"] = GetNewID();
}
row["Code"] = code;
row["Description"] = description;
row["Modified"] = DateTime.Now;
row.AcceptChanges();
}
private int GetNewID()
{
int id = 0;
DataRow[] rows = Table.Select("ID > 0", "ID DESC");
if (rows.Length > 0)
{
id = (int)rows[0]["ID"];
}
return id + 1;
}
private void DataSourceDelete(int id)
{
DataRow[] rows = Table.Select(string.Format("ID={0}", id));
DataRow row = rows[0];
row.Delete();
}
#endregion
#region GridView
private void Bind()
{
GridView1.DataSource = Table;
GridView1.DataBind();
}
#region Editing
protected void GridView1_RowDeleting(object sender, GridViewDeleteEventArgs e)
{
GridView gv = (GridView)sender;
int id = (int)gv.DataKeys[e.RowIndex].Value;
DataSourceDelete(id);
Bind();
}
protected void GridView1_RowEditing(object sender, GridViewEditEventArgs e)
{
//start editing row
GridView gv = (GridView)sender;
gv.EditIndex = e.NewEditIndex;
Bind();
}
protected void GridView1_RowUpdating(object sender, GridViewUpdateEventArgs e)
{
//update editing value
GridView gv = (GridView)sender;
//get id - row key
int id = (int)gv.DataKeys[e.RowIndex].Value;
GridViewRow gRow = gv.Rows[e.RowIndex];
string code = ((TextBox)(gRow.Cells[0].Controls[0])).Text;
string description = ((TextBox)(gRow.Cells[1].Controls[0])).Text;
//write update to datasource
DataSourceUpdate(id, code, description);
//show data
gv.EditIndex = -1;
Bind();
}
protected void GridView1_RowCancelingEdit(object sender, GridViewCancelEditEventArgs e)
{
//cancel editing without saving
GridView gv = (GridView)sender;
gv.EditIndex = -1;
Bind();
}
#endregion
#region New
protected void BtnNew_Click(object sender, EventArgs e)
{
TxtCode.Text = "";
TxtDescription.Text = "";
PnlNew.Visible = true;
BtnNew.Enabled = false;
}
protected void BtnNewSave_Click(object sender, EventArgs e)
{
string code = TxtCode.Text;
string description = TxtDescription.Text;
DataSourceUpdate(code, description);
Bind();
PnlNew.Visible = false;
BtnNew.Enabled = true;
}
protected void BtnNewCancel_Click(object sender, EventArgs e)
{
PnlNew.Visible = false;
BtnNew.Enabled = true;
}
#endregion
#region Sorting
protected void GridView1_Sorting(object sender, GridViewSortEventArgs e)
{
if (string.IsNullOrEmpty(e.SortExpression) == false)
{
if (GvSortDirection == SortDirection.Ascending)
GvSortDirection = SortDirection.Descending;
else
GvSortDirection = SortDirection.Ascending;
GridView gv = (GridView)sender;
SortGridView(gv, e.SortExpression, GvSortDirection);
}
}
//memory sorting direction
private SortDirection GvSortDirection
{
get
{
if (ViewState["SD"] == null)
ViewState["SD"] = SortDirection.Descending;
return (SortDirection)ViewState["SD"];
}
set { ViewState["SD"] = value; }
}
private void SortGridView(GridView gv, string sortExpression, SortDirection direction)
{
DataView dv = new DataView(Table);
dv.Sort = sortExpression + (direction == SortDirection.Ascending ? " ASC" : " DESC");
gv.DataSource = dv;
gv.DataBind();
}
#endregion
#endregion
#region Button
protected void BtnReload_Click(object sender, EventArgs e)
{
LoadDataSource();
}
protected void BtnRefresh_Click(object sender, EventArgs e)
{
Bind();
}
#endregion
}