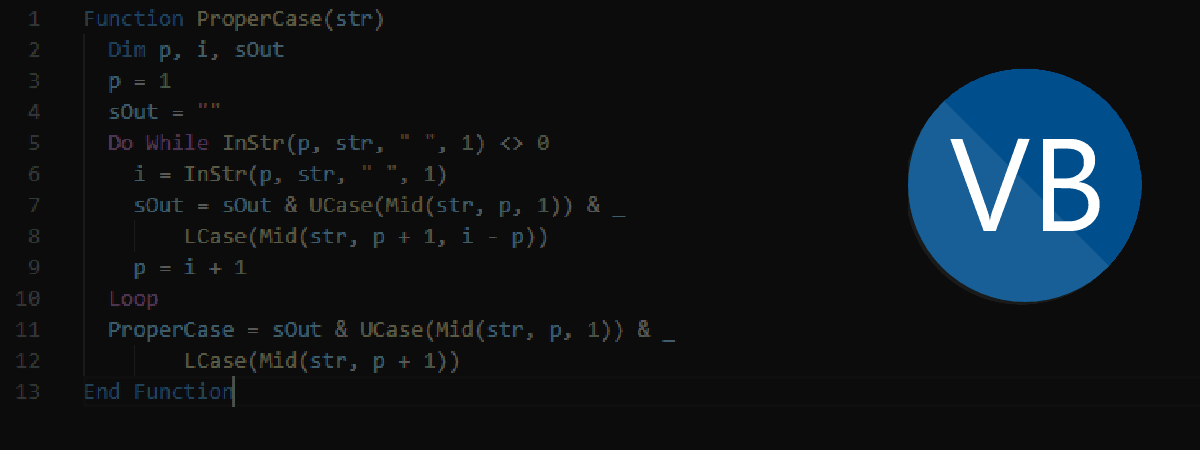
GridView editing e sorting - VB.NET
Il codice in VB.NET dell'esempio GridView editing e sorting in C#
XML: Default.aspx
<%@ Page Language="VB" AutoEventWireup="false" CodeFile="Default.aspx.vb" Inherits="_Default" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml" >
<head id="Head1" runat="server">
<title>GridView Editing example VB.NET - Sgart.it</title>
</head>
<body>
<form id="form1" runat="server">
<h3>GridView Editing example VB.NET - Sgart.it</h3>
<asp:Panel ID="PnlGrid" runat="server">
<asp:GridView ID="GridView1" runat="server" AutoGenerateColumns="False" CellPadding="2"
ForeColor="Black" GridLines="None"
BackColor="LightGoldenrodYellow" BorderColor="Tan" BorderWidth="1px"
DataKeyNames="ID"
OnRowDeleting="GridView1_RowDeleting"
OnRowEditing="GridView1_RowEditing"
OnRowUpdating="GridView1_RowUpdating"
OnRowCancelingEdit="GridView1_RowCancelingEdit"
OnSorting="GridView1_Sorting"
AllowSorting="True" >
<Columns>
<asp:TemplateField ShowHeader="True" HeaderText="Codice" SortExpression="Code">
<ItemTemplate>
<asp:LinkButton ID="LinkButton1" runat="server" CausesValidation="False" CommandName="Select"
Text='<%# Eval("Code") %>'></asp:LinkButton>
</ItemTemplate>
<EditItemTemplate>
<asp:TextBox ID="TxtC" runat="server" Text='<%# Bind("Code") %>'></asp:TextBox>
</EditItemTemplate>
</asp:TemplateField>
<asp:BoundField DataField="Description" HeaderText="Descrizione" SortExpression="Description" />
<asp:BoundField DataField="Modified" HeaderText="Modificato il" ReadOnly="True" SortExpression="Modified" />
<asp:CommandField ShowEditButton="True" ShowDeleteButton="True" HeaderText="Edit" />
</Columns>
<FooterStyle BackColor="Tan" />
<PagerStyle BackColor="PaleGoldenrod" ForeColor="DarkSlateBlue" HorizontalAlign="Center" />
<SelectedRowStyle BackColor="DarkSlateBlue" ForeColor="GhostWhite" />
<HeaderStyle BackColor="Tan" Font-Bold="True" />
<AlternatingRowStyle BackColor="PaleGoldenrod" />
</asp:GridView>
</asp:Panel>
<asp:Panel ID="PnlButton" runat="server">
<asp:Button ID="BtnNew" runat="server" Text="Nuovo" OnClick="BtnNew_Click" />
<asp:Button ID="BtnRefresh" runat="server" Text="Refresh" OnClick="BtnRefresh_Click" />
<asp:Button ID="BtnReload" runat="server" Text="Reload" OnClick="BtnReload_Click" />
</asp:Panel>
<asp:Panel ID="PnlNew" runat="server">
<h4>
Insert</h4>
Code:
<asp:TextBox ID="TxtCode" runat="server"></asp:TextBox>
<br />
Description:
<asp:TextBox ID="TxtDescription" runat="server"></asp:TextBox>
<br />
<asp:Button ID="BtnNewSave" runat="server" OnClick="BtnNewSave_Click" Text="Salva" />
<asp:Button ID="BtnNewCancel" runat="server" Text="Annulla" OnClick="BtnNewCancel_Click" />
</asp:Panel>
<asp:Label ID="LblPostCount" runat="server" Text="Label"></asp:Label>
</form>
</body>
</html>
Visual Basic .NET: Default.aspx.vb
Imports System.Data
Partial Class _Default
Inherits System.Web.UI.Page
Protected Sub Page_Load(ByVal sender As Object, ByVal e As System.EventArgs) Handles Me.Load
If Page.IsPostBack = False Then
LoadDataSource()
PnlNew.Visible = False
End If
End Sub
Public Property Table() As DataTable
Get
Return DirectCast(ViewState("tbl"), DataTable)
End Get
Set(ByVal value As DataTable)
ViewState("tbl") = value
End Set
End Property
Public Property PostCount() As Integer
Get
If Session("count") Is Nothing Then
Session("count") = 0
End If
Return DirectCast(Session("count"), Integer)
End Get
Set(ByVal value As Integer)
Session("count") = value
End Set
End Property
Private Sub IncrasePostCount()
PostCount = PostCount + 1
LblPostCount.Text = "Post Count: " & PostCount.ToString()
End Sub
Public Sub LoadDataSource()
PostCount = -1
IncrasePostCount()
Dim tbl As DataTable = New DataTable()
Dim cols As DataColumnCollection = tbl.Columns
cols.Add("ID", GetType(System.Int32))
cols.Add("Code", GetType(System.String))
cols.Add("Description", GetType(System.String))
cols.Add("Modified", GetType(System.DateTime))
Dim rows As DataRowCollection = tbl.Rows
rows.Add(New Object() {2, "KJ7", "Piastra madre", DateTime.Now})
rows.Add(New Object() {17, "FH5", "Processore", DateTime.Now})
rows.Add(New Object() {4, "ER4", "Ram", DateTime.Now})
rows.Add(New Object() {33, "W34", "Rom", DateTime.Now})
rows.Add(New Object() {1, "SS2", "Hard Disk", DateTime.Now})
rows.Add(New Object() {7, "SD3", "Video", DateTime.Now})
rows.Add(New Object() {8, "TA9", "Tastiera", DateTime.Now})
Table = tbl
Bind()
End Sub
Public Sub DataSourceUpdate(ByVal id As Integer, ByVal code As String, ByVal description As String)
Dim row As DataRow
If ID <> 0 Then
Dim rows As DataRow() = Table.Select(String.Format("ID={0}", ID))
row = rows(0)
Else
row = Table.NewRow()
Table.Rows.Add(row)
row("ID") = GetNewID()
End If
row("Code") = code
row("Description") = description
row("Modified") = DateTime.Now
row.AcceptChanges()
End Sub
Private Function GetNewID() As Integer
Dim id As Integer = 0
Dim rows As DataRow() = Table.Select("ID > 0", "ID DESC")
If (rows.Length > 0) Then
id = DirectCast(rows(0)("ID"), Integer)
End If
Return id + 1
End Function
Private Sub DataSourceDelete(ByVal id As Integer)
Dim rows As DataRow() = Table.Select(String.Format("ID={0}", id))
Dim row As DataRow = rows(0)
row.Delete()
End Sub
Private Sub Bind()
GridView1.DataSource = Table
GridView1.DataBind()
End Sub
Protected Sub GridView1_RowDeleting(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.GridViewDeleteEventArgs)
Dim gv As GridView = DirectCast(sender, GridView)
IncrasePostCount()
If gv.Rows.Count > 0 Then
Try
Dim id As Integer = DirectCast(gv.DataKeys(e.RowIndex).Value, Integer)
DataSourceDelete(id)
Bind()
Catch
End Try
End If
End Sub
Protected Sub GridView1_RowEditing(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.GridViewEditEventArgs)
'start editing row
Dim gv As GridView = DirectCast(sender, GridView)
gv.EditIndex = e.NewEditIndex
Bind()
End Sub
Protected Sub GridView1_RowUpdating(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.GridViewUpdateEventArgs)
'update editing value
Dim gv As GridView = DirectCast(sender, GridView)
'get id - row key
Dim id As Integer = DirectCast(gv.DataKeys(e.RowIndex).Value, Integer)
Dim gRow As GridViewRow = gv.Rows(e.RowIndex)
Dim code As String = DirectCast(gRow.Cells(0).FindControl("TxtC"), TextBox).Text
Dim description As String = DirectCast(gRow.Cells(1).Controls(0), TextBox).Text
'write update to datasource
DataSourceUpdate(id, code, description)
'show data
gv.EditIndex = -1
Bind()
End Sub
Protected Sub GridView1_RowCancelingEdit(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.GridViewCancelEditEventArgs)
'cancel editing without saving
Dim gv As GridView = DirectCast(sender, GridView)
gv.EditIndex = -1
Bind()
End Sub
Protected Sub GridView1_Sorting(ByVal sender As Object, ByVal e As System.Web.UI.WebControls.GridViewSortEventArgs)
If String.IsNullOrEmpty(e.SortExpression) = False Then
If GvSortDirection = SortDirection.Ascending Then
GvSortDirection = SortDirection.Descending
Else
GvSortDirection = SortDirection.Ascending
End If
Dim gv As GridView = DirectCast(sender, GridView)
SortGridView(gv, e.SortExpression, GvSortDirection)
End If
End Sub
'memory sorting direction
Private Property GvSortDirection() As SortDirection
Get
If ViewState("SD") = Nothing Then
ViewState("SD") = SortDirection.Ascending
End If
Return DirectCast(ViewState("SD"), SortDirection)
End Get
Set(ByVal value As SortDirection)
ViewState("SD") = value
End Set
End Property
Private Sub SortGridView(ByVal gv As GridView, ByVal sortExpression As String, ByVal direction As SortDirection)
Dim dv As DataView = New DataView(Table)
If direction = SortDirection.Ascending Then
dv.Sort = sortExpression & " ASC"
Else
dv.Sort = sortExpression & " DESC"
End If
gv.DataSource = dv
gv.DataBind()
End Sub
Protected Sub BtnNew_Click(ByVal sender As Object, ByVal e As System.EventArgs)
TxtCode.Text = ""
TxtDescription.Text = ""
PnlNew.Visible = True
BtnNew.Enabled = False
End Sub
Protected Sub BtnRefresh_Click(ByVal sender As Object, ByVal e As System.EventArgs)
Bind()
End Sub
Protected Sub BtnReload_Click(ByVal sender As Object, ByVal e As System.EventArgs)
LoadDataSource()
End Sub
Protected Sub BtnNewSave_Click(ByVal sender As Object, ByVal e As System.EventArgs)
Dim code As String = TxtCode.Text
Dim description As String = TxtDescription.Text
DataSourceUpdate(0, code, description)
Bind()
PnlNew.Visible = False
BtnNew.Enabled = True
End Sub
Protected Sub BtnNewCancel_Click(ByVal sender As Object, ByVal e As System.EventArgs)
PnlNew.Visible = False
BtnNew.Enabled = True
End Sub
End Class